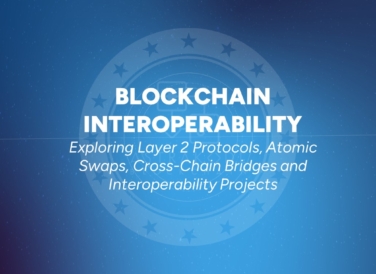
Blockchain Interoperability: Exploring Layer 2 Protocols, Atomic Swaps, Cross-Chain Bridges and Interoperability Projects
In the dynamic world of blockchain technology, the concept of blockchain interoperability stands as a…
Blockchain technology has revolutionized the digital landscape, offering decentralized systems that ensure transparency, immutability, and trust. At the heart of this ecosystem are smart contracts — self-executing agreements with the terms directly embedded in code. Platforms like Ethereum have harnessed the power of smart contracts to automate transactions across various sectors, from finance to supply chains.
However, the very attributes that make smart contracts powerful — immutability and automation — also introduce significant risks. Once deployed, these contracts cannot be modified, leaving any vulnerabilities in the code open to exploitation. In an environment where contracts often manage substantial financial assets, a single flaw can result in catastrophic losses.
This is where auditing becomes indispensable. Smart contract auditing is a systematic process of reviewing code to identify and mitigate security vulnerabilities. It ensures that the contract operates as intended without exposing users or assets to potential risks. Auditing not only protects developers and users from financial and reputational damage but also strengthens the overall blockchain ecosystem by promoting trust and reliability.
In the world of blockchain development, Solidity has emerged as the dominant language for creating smart contracts on Ethereum. Its ability to encode complex operations and facilitate automated execution has made it a preferred choice for developers. However, with great power comes great responsibility. The security of Solidity contracts is paramount, as even minor coding oversights can lead to vulnerabilities with devastating consequences. This is where auditing plays a critical role.
Auditing in Solidity development goes beyond simply checking for bugs — it’s about safeguarding the integrity of the entire contract. Solidity contracts often manage valuable digital assets or control critical processes. Without rigorous auditing, these contracts can become gateways for malicious attacks or operational failures.
Auditing frequently uncovers vulnerabilities that could otherwise lead to catastrophic failures or exploits. Some of the most prevalent issues include:
checks-effects-interactions
pattern or Solidity’s nonReentrant
modifier from ReentrancyGuard
.onlyOwner
function modifier is not used correctly, attackers could gain access to critical operations.Auditing not only identifies these vulnerabilities but also provides actionable recommendations to address them. By integrating audits into the development lifecycle, Solidity developers can ensure their contracts are robust, efficient, and secure. This proactive approach builds trust within the blockchain ecosystem and helps pave the way for broader adoption of decentralized technologies.
Libraries play a crucial role in Solidity development, acting as reusable blocks of code that simplify complex tasks and promote modular, efficient, and secure smart contract design. By abstracting common functionality into libraries, developers can focus on building robust and scalable contracts without reinventing the wheel. However, as beneficial as they are, libraries can also become a source of vulnerabilities if misused.
In Solidity, libraries are collections of functions designed to operate on specific data types or perform repetitive tasks. Unlike regular contracts, libraries cannot hold state or receive Ether, making them lightweight and gas-efficient tools for enhancing smart contract functionality.
Key advantages of libraries include:
Several libraries have become industry standards due to their reliability and security:
A recent empirical study analyzing over 1,000 smart contracts uncovered 905 cases of library misuse across 456 contracts, emphasizing the need for developers to be vigilant. By identifying patterns of misuse and their consequences, this research provides a roadmap for safer and more efficient use of libraries in smart contracts.
The study categorized library misuse into eight patterns, ranging from errors in development to flaws in implementation. These issues often stem from a lack of security awareness, overestimation of library functionality, or negligence in keeping libraries updated. Notably:
Access the full research study: Revealing Hidden Threats: An Empirical Study of Library Misuse in Smart Contracts
Libraries like SafeMath provide essential tools for enhancing security in Solidity smart contracts by wrapping critical functions with additional checks. However, these wrappers must be implemented correctly to prevent vulnerabilities or unintended behaviors.
add
and sub
, were widely used to prevent integer overflows and underflows before Solidity 0.8 introduced built-in arithmetic checks. Developers sometimes mistakenly mix native arithmetic operations with SafeMath, leading to inconsistent logic and bypassed checks.using SafeMath for uint256;
uint256 result = value1.add(value2);
safeApprove
or inconsistently applying SafeMath nullifies their intended security benefits, opening the contract to vulnerabilities. Developers must adhere to best practices to ensure the integrity of their smart contracts.Developers sometimes rely too heavily on libraries without understanding their limitations, leading to vulnerabilities when the library functions fail to perform as assumed.
Address.isContract
function was used to block contract interactions. However, it couldn’t detect contracts with only constructors, enabling attackers to bypass restrictions using flash loans.Conversely, developers may overlook the full functionality of a library, resulting in redundant or conflicting code.
getAmountIn
function to calculate inputs and outputs for token exchanges. They ignored the function’s handling fee logic, causing incorrect return values.One of the most common misuse patterns occurs when developers adopt a library like SafeMath but fail to replace all relevant operations with the library’s secure functions.
Despite newer and more secure versions of libraries being available, many smart contracts continue to rely on outdated versions, perpetuating vulnerabilities that have already been addressed. This lack of adoption not only exposes contracts to unnecessary risks but also undermines efforts to standardize secure practices across the ecosystem.
Example: Outdated Usage of SafeERC20’s safeApprove
Function
A notable instance of outdated library usage involves the safeApprove
function in earlier versions of the SafeERC20 library. This function was used to manage token allowances but was inherently risky due to its potential for race conditions. As a result, it was deprecated in favor of safeIncreaseAllowance
and safeDecreaseAllowance
, which eliminate the race condition by incrementally adjusting allowances.
However, despite the availability of these safer alternatives, some developers still use older versions of SafeERC20 that include the deprecated safeApprove
function. This introduces vulnerabilities into their contracts that could easily be avoided by upgrading to the latest library version.
safeIncreaseAllowance
and safeDecreaseAllowance
to securely adjust allowances, as shown:IERC20(token).safeIncreaseAllowance(spender, amountToIncrease);
IERC20(token).safeDecreaseAllowance(spender, amountToDecrease);
By using updated library versions and adhering to best practices, developers can mitigate security risks and ensure that their contracts remain robust and compliant with current standards.
With the introduction of native overflow checks in Solidity 0.8, the use of SafeMath became unnecessary. However, many contracts continued to use it, resulting in increased gas costs. One contract invoking SafeMath over 1,000 times wasted over 1,123,500 gas units.
The patterns of library misuse highlight the importance of developer education and best practices. By understanding the capabilities and limitations of libraries, maintaining updated dependencies, and adopting comprehensive audits, developers can avoid these pitfalls. Addressing library misuse is not just a matter of security—it’s a step toward building a more efficient and trustworthy blockchain ecosystem.
As blockchain applications grow in complexity, the importance of secure and efficient Solidity smart contracts has reached new heights. Auditing practices are evolving to address modern challenges, with trends like AI-driven tools, gas optimization, and holistic analysis taking center stage. These innovations aim to enhance security while making auditing more accessible to developers.
Artificial intelligence is transforming smart contract auditing by automating vulnerability detection and adapting to emerging threats. AI tools can quickly identify issues like reentrancy vulnerabilities, gas inefficiencies, and insecure external calls, reducing the burden on developers. Machine learning models enhance these tools by learning from past vulnerabilities and predicting potential risks, enabling a proactive approach to security.
With Ethereum undergoing a series of upgrades aimed at improving scalability and reducing transaction costs, the need for gas optimization has evolved. While the blockchain’s roadmap promises significant improvements, optimizing contract efficiency remains essential — particularly for large-scale applications where gas consumption can still have a significant impact. Modern audits focus on refining computationally expensive operations, reducing redundant calculations, and optimizing storage usage. These practices ensure that contracts remain cost-effective and scalable, contributing to a smoother user experience even as the underlying infrastructure improves.
Effective auditing combines static analysis (reviewing code structure for flaws) with dynamic analysis (simulating real-world interactions to identify runtime vulnerabilities). This holistic approach ensures that contracts are secure throughout their lifecycle, from development to deployment.
Among the innovative tools aligned with these trends, AstraKode Smart Contract Auditor stands out. It integrates AI-powered insights with both static and dynamic analysis, enabling comprehensive vulnerability detection. AstraKode simplifies the auditing process with a user-friendly interface, making it accessible to developers of all experience levels. Its focus on gas optimization ensures contracts are not only secure but also cost-effective.
By automating much of the manual effort involved in auditing and offering actionable suggestions, AstraKode helps developers address security challenges efficiently while staying ahead of emerging risks.
Smart contract auditing is an essential pillar of blockchain security, ensuring that the automated systems powering decentralized applications are both reliable and secure. The immutable nature of blockchain makes proactive auditing critical, as vulnerabilities left unaddressed can result in devastating consequences, from financial losses to a breakdown in user trust.
By identifying and mitigating common issues like reentrancy attacks, gas inefficiencies, and access control flaws, audits serve as a safeguard for developers and users alike. Additionally, the evolving use of libraries in Solidity highlights the need for careful implementation and regular updates to avoid misuse. The rise of AI-driven tools, such as AstraKode Smart Contract Auditor, further underscores the potential for innovation to simplify and enhance the auditing process, making it more accessible and effective for developers at all levels.
As the blockchain ecosystem grows, developers must prioritize secure coding practices. This includes integrating auditing into the development lifecycle, staying updated with the latest tools and trends, and committing to continuous learning and improvement. By doing so, developers not only protect their projects but also contribute to the broader effort of creating a more secure and trustworthy blockchain ecosystem.
The future of blockchain depends on robust security practices. Developers hold the key to this future by ensuring their smart contracts are thoroughly audited and resilient against evolving threats. Let’s build a safer, more reliable decentralized world together.
In the dynamic world of blockchain technology, the concept of blockchain interoperability stands as a…
A Digital ID is an electronic form of identification that allows individuals to prove who…
In the vibrant landscape of blockchain technology, smart contracts have emerged as a game-changing innovation,…